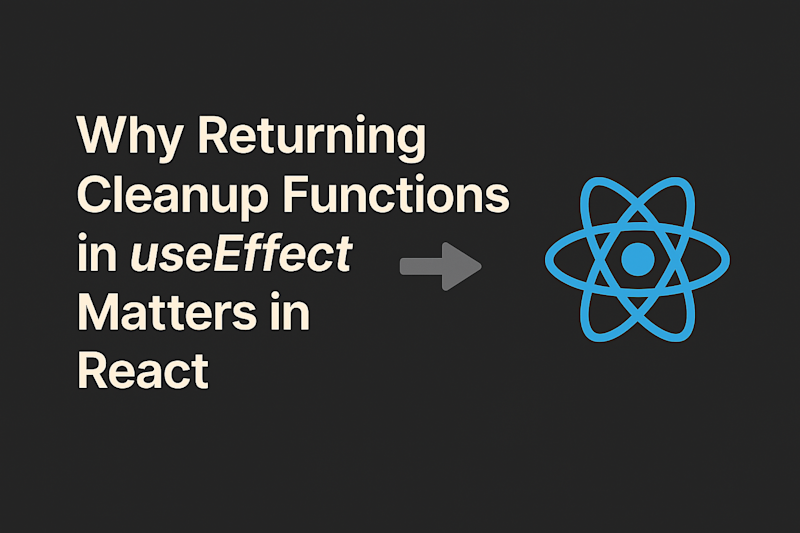
Why Returning Cleanup Functions in useEffect Matters in React
React’s useEffect
hook is one of the most powerful and commonly used features in functional components. It enables side effects like data fetching, subscriptions, timers, and direct DOM manipulation. But with great power comes great responsibility. One often-overlooked aspect of useEffect
is the importance of returning a cleanup function—especially when working with timers, event listeners, or subscriptions.
In this post, we'll explore:
- What
useEffect
is and its primary use - The concept and importance of cleanup functions
- A real-world example involving
setTimeout
- Differences in behavior between development and production
- A step-by-step guide to adding cleanup functions
- Best practices to follow for safe, maintainable code
What is useEffect
?
useEffect
is a React Hook introduced in version 16.8 that lets you perform side effects in function components.
Its Primary Purpose
The useEffect
hook runs code after the component renders. You can use it to:
- Fetch data from APIs
- Set up event listeners
- Update the DOM manually
- Start timers or intervals
Here’s a basic example:
import { useEffect } from "react";
function MyComponent() {
useEffect(() => {
console.log("Component mounted");
// Optional cleanup function
return () => {
console.log("Component will unmount");
};
}, []); // Empty dependency array = run once on mount
}
What is a Cleanup Function?
A cleanup function is the optional return value from your useEffect
callback. It allows you to tear down or cancel any lingering side effects before the component unmounts or the effect runs again.
Why It Matters
Without cleanup:
- Event listeners may pile up
- API requests may resolve after a component is unmounted
- Timers may fire on stale or non-existent components
- Memory leaks and buggy behavior become more likely
A Common Pitfall: Forgetting to Clear a setTimeout
Let’s say you're showing a notification that disappears after 3 seconds:
import { useEffect, useState } from "react";
function Notification() {
const [visible, setVisible] = useState(true);
useEffect(() => {
const timer = setTimeout(() => {
setVisible(false);
}, 3000);
}, []);
return visible ? <div>You've got mail!</div> : null;
}
What Can Go Wrong?
If the component unmounts before the timeout completes, the setVisible(false)
will run on an unmounted component. In development, React warns you. In production, you may see:
- Silent failures
- State updates on unmounted components
- Flashing UI
- Unintended behavior
Why Behavior Differs in Development vs Production
React’s Strict Mode (enabled in development) intentionally calls some lifecycle methods twice to help catch side effects and improper cleanup. But in production, this behavior is skipped for performance.
So:
- In development, you might see the
setTimeout
run twice. - In production, it might “work” but cause subtle bugs or memory leaks.
How to Fix It: Adding a Cleanup Function
Here’s how to safely handle the timer:
import { useEffect, useState } from "react";
function Notification() {
const [visible, setVisible] = useState(true);
useEffect(() => {
const timer = setTimeout(() => {
setVisible(false);
}, 3000);
// Cleanup function
return () => {
clearTimeout(timer);
};
}, []);
return visible ? <div>You've got mail!</div> : null;
}
Step-by-Step Breakdown:
- Start the timer with
setTimeout
. - Return a function from
useEffect
that clears the timer. - When the component unmounts or re-renders (if dependencies changed), React automatically calls the cleanup function.
Benefits of Returning Cleanup Functions
- 🧼 Prevent memory leaks
- 🔥 Cancel stale async actions or timers
- 🧠 Avoid state updates on unmounted components
- ⚙️ Ensure consistent behavior across environments
- 💪 Make your components more predictable and testable
Best Practices for useEffect
Cleanup Functions
- Always clean up async side effects (timers, intervals, fetches, etc.)
- Remove event listeners like
window.addEventListener
inside the cleanup. - Abort fetch requests using
AbortController
where possible. - Use the cleanup function even if the effect runs only once—you never know when future changes might introduce bugs.
- Keep your
useEffect
logic focused: One concern per effect. Break it up if needed.
Conclusion
The useEffect
hook is an indispensable part of writing modern React apps, but it comes with responsibility. Not returning a cleanup function when needed can lead to bugs, inconsistent behavior, and memory leaks—especially when dealing with timers, subscriptions, or asynchronous tasks.
By taking the time to understand and implement proper cleanup logic, you make your codebase more robust, reliable, and maintainable.
TL;DR
✅ Always return a cleanup function in useEffect
when working with side effects like timers or listeners.
💥 It prevents memory leaks, weird bugs, and unexpected behavior.
🧠 Write clean effects for clean components.
📦 CodeSandbox Demo
See the working example here:
👉 https://codesandbox.io/p/sandbox/jtkg8q